Let's Code Kotlin: For & While
Fors and Whiles in Kotlin are pretty similar to their Java counterparts. Let's go over them so you can become proficient in Kotlin!
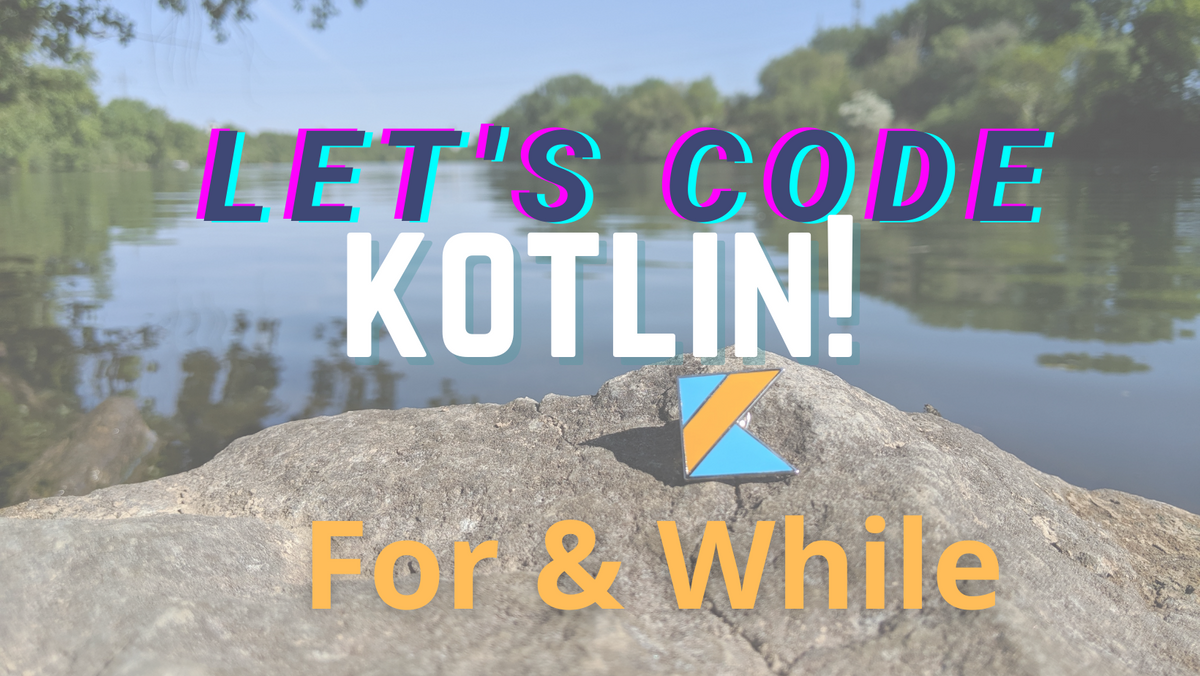
Last week I went over If, Else, And When. This week, I explore two more pieces of Kotlin syntax. Lucky for us, they are almost equivalent to their Java counterparts: For
and While
.
For
You can loop through a collection in Kotlin similar to how you can in Java.
for (spell in spellbook) {
print("${spell.name}")
}
If you don't need anything more advanced, this will work for you just fine in many cases without any added complexity. Actually, you can perform this version of looping in another way by making use of a convenient extension function on Collection: forEach
.
spellbook.forEach {
print("${it.name}")
}
spellbook.forEach { spell ->
print("${spell.name}")
}
In the first example,it
is the default name for each item in the collection. The second example shows you how to rename it
to something a bit more specific.
Looping through a range of numbers is a bit different. You may be used to writing for (int i = 0; i < 10, i++)
. That's not a complex thing to write, but it's much simpler in Kotlin.
for (i in 0..10) {
// this will loop, from 0 to 12, inclusive.
}
Despite being simpler, however, I find that I almost never loop through things like this. In Java, it was customary to start at 0, go until the size of the list, incrementing by one each time. In the code block of that for loop, I'd make a variable for the item at the given index. I've abandoned this method in Kotlin. I think I've used it maybe once; I find myself using the .forEach
extension method more.
It's worth noting that ranges can get complex. They're not too difficult and open up some possibilities that could be useful, like looping from ten to zero but only going through the even indices. Again, I don't loop through ranges anymore, but it's interesting to see how this differs from its Java implementation.
for (i in 10 downTo 0 step 2) {
// this will loop from 10 to 0, but only the event numbers, inclusive
}
You can find more documentation on For loops on the official Kotlin page (and also the page about Ranges).
While
For the sake of completion, while
and do while
are pretty much exactly like they are in Java.
while (x > 10) {
x += 2
}
do {
update()
) while (x != 3)
Short and simple! I've created a page that documents things I find out about Kotlin. Check that out, and stay tuned for more Kotlin tips!