Let's Code Kotlin: If, Else, When
Kotlin is very similar to Java, but a refresher is also good to have. This week, I go over If, Else, and When.
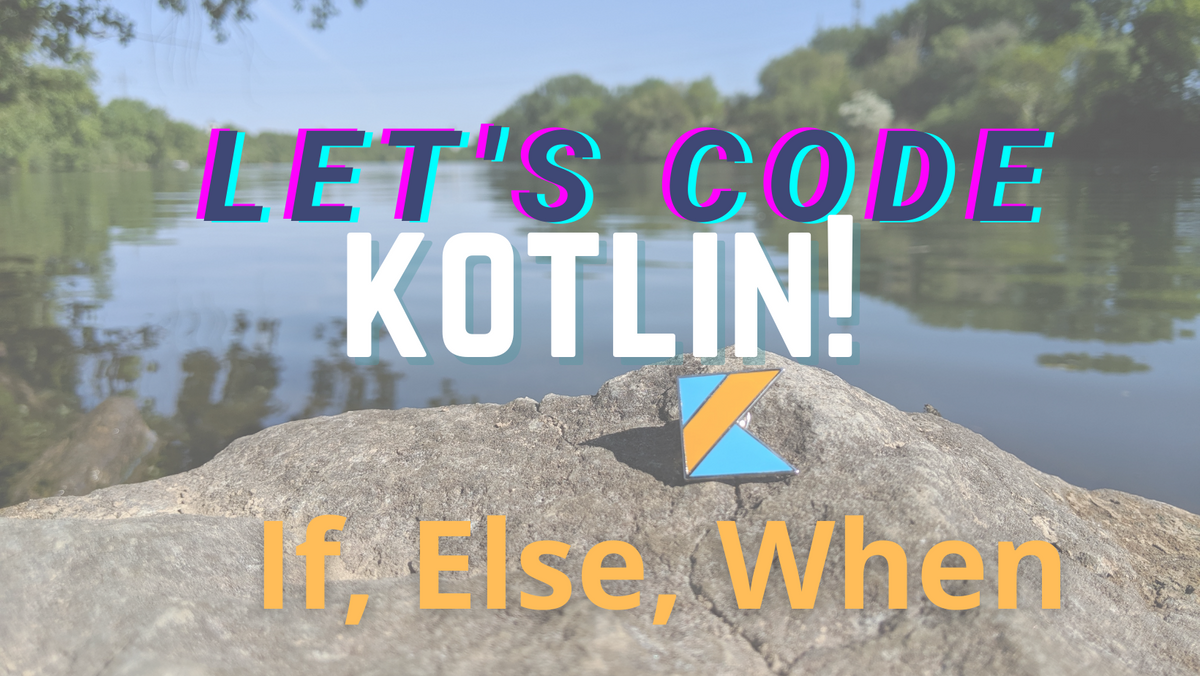
Kotlin was an easy language to switch to, with a very small learning curve. Most of it looks similar to it's Java counterpart, yet somehow easier. To go along with the other posts I have about switching from Java to Kotlin, today I'll go over a few basics just to make sure it's clear how easy the switch is: if
s, else
s, and when
s.
If & Else
Overall, Ifs and Elses don't change much. Outside of leaving off semicolons, you can use your knowledge of Java to watch if-else statements.
if (makeIntimidationCheck() >= DC)
// intimidation success code
var proficiencyBonus: Int
if (level >= 17) {
proficiencyBonus = 6
} else if (level >= 13) {
proficiencyBonus = 5
} else if (level >= 9) {
proficiencyBonus = 4
} else if (level >= 5) {
proficiencyBonus = 3
} else {
proficiencyBonus = 2
}
It's worth noting that there is no ternary operator in Kotlin, meaning you can't do var value = isSomething ? valueOne : valueTwo
. That kinda makes me sad (I like the aesthetic, sue me), but an if-else can handle it just fine the same way.
var charismaSkillBonus = 3
var intimidationBonus = if (isProficientInIntimidation) proficiencyBonus + charismaSkillBonus else charismaSkillBonus
var intimidationBonus = if (isProficientInIntimidation) {
// other stuff
proficiencyBonus + charismaSkillBonus
} else {
// other stuff
charismaSkillBonus
}
When
In Java (and many other languages), you can use a Switch statement. In Kotlin, it's called when
. Let's turn that previous example about proficiencyBonus
into a much better when
:
var proficiencyBonus = when (level) {
1, 2, 3, 4 -> 2
5, 6, 7, 8 -> 3
9, 10, 11, 12 -> 4
13, 14, 15, 16 -> 5
17, 18, 19, 20 -> 6
}
Eh, okay, that's probably not the best. Luckily, in my example, level
can only be a number from 1 to 20. But, knowing this, when can modify that a bit, showing some of the complexities that a when
can handle.
var proficiencyBonus = when (level) {
in 1..4 -> 2
in 5..8 -> 3
in 9..12 -> 4
in 13..16 -> 5
in 17..20 -> 6
}
Awesome! Much better, I think.
A when
can also serve as a more direct replacement for an if-else chain. If you remove the argument, you can do whatever you want.
var proficiencyBonus = when {
level >= 17 -> 6
level >= 13 -> 5
level >= 9 -> 4
level >= 5 -> 3
else -> 2
}
There is some official Kotlin documentation that goes into a bit more depth on these things and more. Check it out and let me know in the comments or on Twitter (@GatlingXYZ) if you have any questions or want me to flesh anything out a bit more. The next post in my "Let's Code" series for Kotlin will be about For
s and While
s, so stay tuned!